会员活跃度分析之ODS建表和数据加载
ODS建表和数据加载
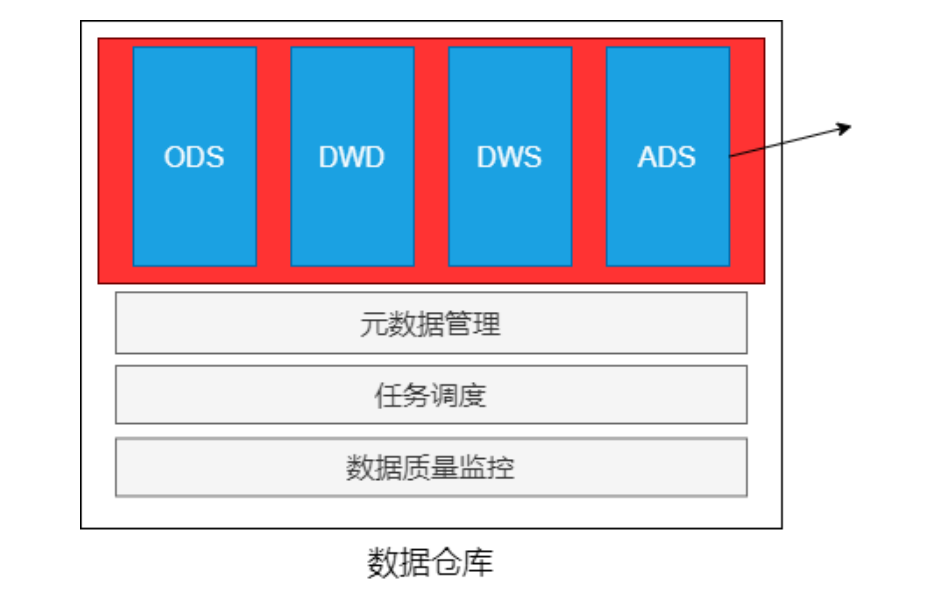
ODS层的数据与源数据的格式基本相同。
创建ODS层表:
1 | use ODS; |
加载启动日志数据:
script/member_active/ods_load_log.sh
可以传参数确定日志,如果没有传参使用昨天日期
1 | #!/bin/bash |
json数据处理
数据文件中每行必须是一个完整的 json 串,一个 json串不能跨越多行。
Hive 处理json数据总体来说有三个办法:
-
使用内建的函数get_json_object、json_tuple
-
使用自定义的UDF
-
第三方的SerDe
使用内建函数处理
-
get_json_object(string json_string, string path)
返回值:String
说明:解析json字符串json_string,返回path指定的内容;如果输入的json字符串无效,那么返回NUll;函数每次只能返回一个数据项;
-
json_tuple(jsonStr, k1, k2, …)
返回值:所有的输入参数、输出参数都是String;
说明:参数为一组键k1,k2,。。。。。和json字符串,返回值的元组。该方法比get_json_object高效,因此可以在一次调用中输入多个键;
explode,使用explod将Hive一行中复杂的 array 或 map 结构拆分成多行。
测试数据格式:
1 | user1;18;male;{"id": 1,"ids": [101,102,103],"total_number": 3} |
建表加载数据:
1 | CREATE TABLE IF NOT EXISTS jsont1( |
json的处理:
1 | -- get 单层值 |
小结:json_tuple 优点是一次可以解析多个json字段,对嵌套结果的解析操作复杂;
使用UDF处理
自定义UDF处理json串中的数组。自定义UDF函数:
-
输入:json串、数组的key
-
输出:字符串数组
pom文件增加依赖
1 | <dependency> |
1 | package cn.lagou.dw.hive.udf; |
使用自定义 UDF 函数:
1 | -- 添加开发的jar包(在Hive命令行中) |
使用SerDe处理
序列化是对象转换为字节序列的过程;反序列化是字节序列恢复为对象的过程;
对象的序列化主要有两种用途:
-
对象的持久化,即把对象转换成字节序列后保存到文件中
-
对象数据的网络传送
SerDe 是Serializer 和 Deserializer 的简写形式。Hive使用Serde进行行对象的序列与反序列化。最后实现把文件内容映射到 hive 表中的字段数据类型。SerDe包括Serialize/Deserilize 两个功能:
-
Serialize把Hive使用的java object转换成能写入HDFS字节序列,或者其他系统能识别的流文件
-
Deserilize把字符串或者二进制流转换成Hive能识别的java object对象
Read : HDFS files => InputFileFormat => <key, value> => Deserializer => Row object
Write : Row object => Seriallizer => <key, value> => OutputFileFormat => HDFS files
常见:https://cwiki.apache.org/confluence/display/Hive/DeveloperGuide#DeveloperGuide-HiveSerDe
Hive本身自带了几个内置的SerDe,还有其他一些第三方的SerDe可供选择。
1 | create table t11( |
LazySimpleSerDe(默认的SerDe)
ParquetHiveSerDe
OrcSerde
对于纯 json 格式的数据,可以使用 JsonSerDe 来处理。
1 | {"id": 1,"ids": [101,102,103],"total_number": 3} |
1 | create table jsont2( |
各种Json格式处理方法小结:
1、简单格式的json数据,使用get_json_object、json_tuple处理
2、对于嵌套数据类型,可以使用UDF
3、纯json串可使用JsonSerDe处理更简单