JavaWeb之Filter过滤器和Listener监听器
Filter过滤器
所谓的过滤.指的是过滤请求.
有时我们进行后段项目开发时,有些请求,需要特定的条件才能操作;我们可以通过Filter,来过滤不满足的用户操作.
例如:
用于的个人中心, 应该是登录 后才可以查看的.
当用户请求个人中心页面时, 我们就可以编写过滤器, 将未登录的所有用户过滤掉, 并重定向至登录页面.
采用了面向切面编程思想(AOP).
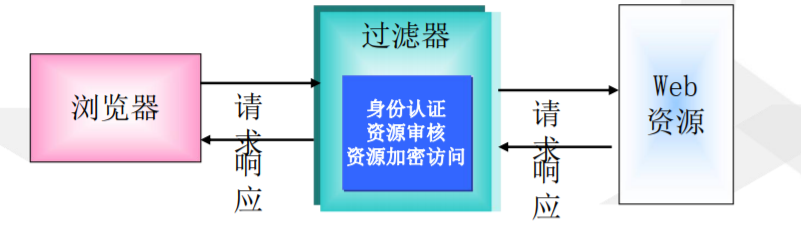
过滤器的使用
编写home.jsp
1 | <%@ page language="java" contentType="text/html; charset=UTF-8" |
1.编写一个类,实现Filter接口
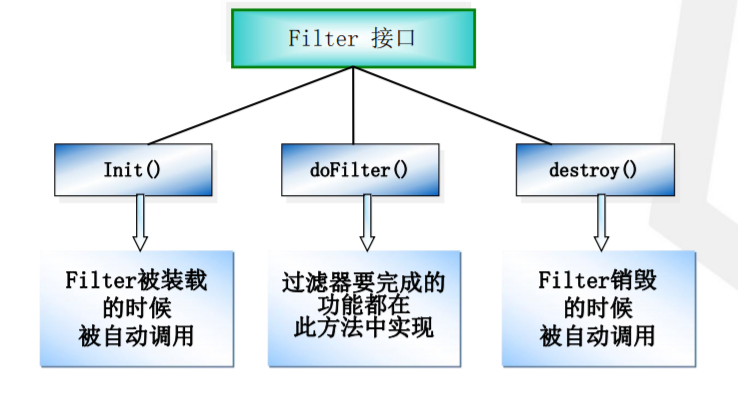
1 | package demo1; |
2.通过web.xml或注解的方式,配置过滤器的过滤地址(home.jsp).
web.xml
1 | <filter> |
注解
1 | 在ParamFilter类的类体上注解@WebFilter("/home.jsp") |
过滤器链
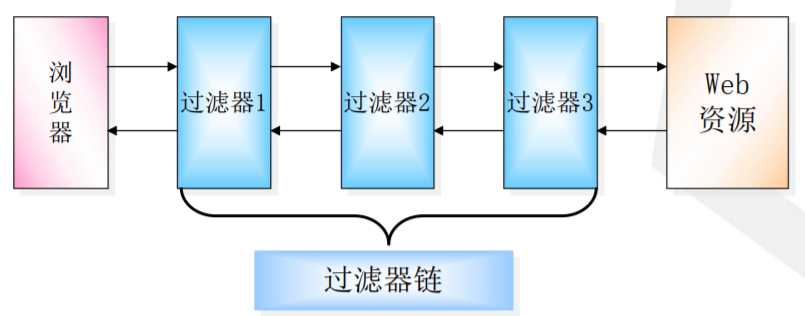
当多个过滤器,过滤地址重复时,就形成了过滤器链.用户的请求,需要过滤器链中的所有过滤器放行,才可以正常访问.
过滤器链执行的顺序:
1 | web.xml中的顺序:按照web.xml中配置的先后顺序, 来执行. |
在web下新建login.jsp,并输入
1 | <body> |
在src下新建loginservlet.class,并输入
1 | import javax.servlet.ServletException; |
在src下新建过滤器CharSetFilter.class,并输入
1 | import javax.servlet.*; |
在src下新建过滤器NullFilter.class,并输入
1 | import javax.servlet.*; |
此时访问login.do是输入表单后,先执行过滤器CharSetFilter,在执行NullFilter,因为是一首字母排序.
如果在web.xml里添加NullFilter的过滤地址,则先执行的是NullFilter
Listener
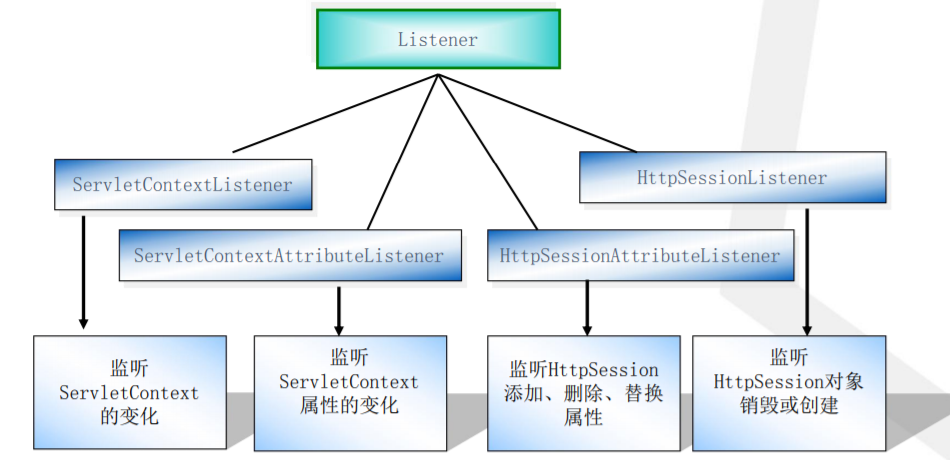
监听器,监听是服务器的一些事件.
两类事件:
1 | 1.服务器中组件的生命周期相关事件. |
-
ServletContextListener
用于监听Servlet上下文的创建与销毁.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
import javax.servlet.annotation.WebListener;
public class MyServletContextListener implements ServletContextListener {
/**
* 当上下文对象即将销毁时,方法执行
* 上下文对象销毁的时机,是服务器关闭或项目被卸载.所以我们常在这里进行全局资源释放的操作.
*/
public void contextDestroyed(ServletContextEvent arg0) {
System.out.println("项目关闭了");
}
/**
* 当上下文对象被创建初始化时,这个方法执行
* 因为上下文对象创建的时机是服务器中项目启动时,所以我们常在这里进行全局资源的初始化操作.
*/
public void contextInitialized(ServletContextEvent arg0) {
System.out.println("项目启动了");
}
} -
ServletContextAttributeListener
用于监听ServletContext中属性的变化
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41import javax.servlet.ServletContextAttributeEvent;
import javax.servlet.ServletContextAttributeListener;
import javax.servlet.annotation.WebListener;
/**
*
*/
public class MyServletContextAttributeListener implements ServletContextAttributeListener {
/**
* 当向上下文中 添加属性时, 代码执行
*/
public void attributeAdded(ServletContextAttributeEvent e) {
//从事件对象中, 得到存储的键和值
String key = e.getName();
Object value = e.getValue();
System.out.println("监听到ServletContext中数据的增加:"+key+" --> "+value);
}
/**
* 当从上下文中 移除属性时, 代码执行
*/
public void attributeRemoved(ServletContextAttributeEvent e) {
//从事件对象中, 得到被移除的键和值
String key = e.getName();
Object value = e.getValue();
System.out.println("监听到ServletContext中数据的移除:"+key+" --> "+value);
}
/**
* 当操作上下文对象 存储数据 ,发生替换时, 代码执行.
*/
public void attributeReplaced(ServletContextAttributeEvent e) {
//从事件对象中, 得到被替换掉的旧的键和值
String key = e.getName();
Object oldValue = e.getValue();
System.out.println("监听到ServletContext中数据的替换, 旧数据:"+key+" --> "+oldValue);
//从事件对象中, 得到上下文对象
Object newValue = e.getServletContext().getAttribute(key);
System.out.println("监听到ServletContext中数据的替换, 新数据:"+key+" --> "+newValue);
}
} -
HttpSessionListener
用于监听session的创建与销毁.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27import javax.servlet.annotation.WebListener;
import javax.servlet.http.HttpSessionEvent;
import javax.servlet.http.HttpSessionListener;
import java.util.ArrayList;
import java.util.Random;
public class MySessionListener implements HttpSessionListener {
private static int count = 0;
private static Random r = new Random();
ArrayList<Integer> nums = new ArrayList<>();
public void sessionCreated(HttpSessionEvent arg0) {
//30-60的随机数字
int num = r.nextInt(31)+30;
count+=num;
nums.add(num);
}
public void sessionDestroyed(HttpSessionEvent arg0) {
int num = nums.remove(nums.size()-1);
count-=num;
}
public static int getCount() {
return count;
}
} -
HttpSessionAttributeListener
用于监听session中的数据的增加 ,删除, 修改.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59import javax.servlet.annotation.WebListener;
import javax.servlet.http.HttpSessionAttributeListener;
import javax.servlet.http.HttpSessionBindingEvent;
import java.util.ArrayList;
/**
*
*/
public class MyHttpSessionAttributeListener implements HttpSessionAttributeListener {
private static ArrayList<Object> data = new ArrayList<>();
public static ArrayList<Object> getData() {
return data;
}
/**
* 当session中存储数据时 , 方法执行
*/
public void attributeAdded(HttpSessionBindingEvent e) {
//获取新增加的数据
String name = e.getName();
Object value = e.getValue();
// 当登录成功时, 通常我们会将用户名存储在session中, 用于会话跟踪.
if("username".equals(name)) {
//当一个用户名存储到session时, 我们就将这个用户名 加入到集合中
data.add(value);
}
System.out.println("新用户登录, 用户名已存储");
}
/**
* 当从session中移除数据时, 方法执行
*/
public void attributeRemoved(HttpSessionBindingEvent e) {
//获取删除的数据
String name = e.getName();
Object value = e.getValue();
if("username".equals(name)) {
//当一个用户名存储到session时, 我们就将这个用户名 加入到集合中
data.remove(value);
}
System.out.println("用户退出登录, 用户名从列表中移除");
}
/**
* 当向session中设置键值对, 由于键重复, 导致值被替换时 , 方法执行
*/
public void attributeReplaced(HttpSessionBindingEvent e) {
//获取被替换的旧数据
String name = e.getName();
Object oldValue = e.getValue();
Object newValue = e.getSession().getAttribute(name);
data.remove(oldValue);
data.add(newValue);
System.out.println("用户更换帐号登录, 用户名从列表中更新");
}
}
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 WeiJia_Rao!